Introduction
There are lots of toolkits for building single page apps or "Native HTML5 Apps" if you will. It seems they're either too generic and unopinionated or too tightly coupled or require a lot of knowledge and understanding of the framework. After having built lots of single page apps with various tools at &yet we've surmised that the ideal seems to lie somewhere in the middle.
Ultimately we'd like something that is comprised of lots of independent little tools that each to one thing (see Unix philosophy).
That said, starting with a group of completely unassembled little pieces is inefficient because there are lots of patterns common to most apps. So we need an opinionated starting point.
Enter HumanJS. It's not a framework per se, it's a bag of pre-assembled little tools that you're free to rip apart and add to with the following goals in mind.
Goals
- Provides an opinionated starting point for an app
- Easy to get up and running
- Minimal framework-specific knowledge required (knowing JavaScript well gets you 95% there)
- Minimal magic
- Solve the develpment workflow problems out of the box:
- Unminified and re-built automatically in develpment
- Minfied, cached for production use
- Switching between the two with a single config flag
- Use node.js to simplify develpment
- 100% client rendered
- Readability
- App can be served as static content by any server (node.js not required in production)
- Easy to collaborate on:
- Clear file structure where everything has a logical place
- Proper seperation of concerns
- Use npm + browserify for package management but still play nicely with non-common JS libraries.
Quick Start Guide
- install node.js
-
install humanjs —
$ npm install humanjs -g
-
run
humanjs
to create your new app folder, build your project scaffolding, and run your app.$ humanjs
-
open your browser to:
http://localhost:3000
- that's it!
What's included?
- Well-architected shell of a single page app to serve as a starting point for your app.
- A solution for using jade templates and templatizer to pre-compile client templates.
- A fully functional development environment that will watch for changes. You just modify and refresh (without manual build steps).
- Changing one flag in your config puts it in production mode which builds and serves minfied, uniquely named, and permanently cachable static files.
- A clientside routing system using HTML5 pushState.
- A main layout template is rendered by the main view that includes a page container where page views are rendered as the user navigates to different URLs in the app.
- An example of rendering a collection of models in a container.
- A mock REST API to demonstrate how you might talk to an API to fetch data.
- A solution for declaritive binding of model properties to views, that is completely decoupled from the template engine. See human-view below for more details.
- A solution for creating readable, type-checked, and very explicitly defined models. This is hugely important in team enviroments where you need somewhere to reference what is being stored on your models. See human-model for more detail.
Human JavaScript book
A 100+ page e-book providing additional context, explanations and philosophy behind the tools documented here is available for $39.
@gendoc human-model ../human-model/README.md @gendoc human-view ../human-view/README.mdRouter
HumanJS just uses Backbone's router so just see Backbone's router docs for more info.
Moonboots
Moonboots is a tool to help you intelligently deal with different goals of serving JS during development and in production.
In development we explicitly don't want to cache or minify our JS and CSS files while working. We want to organize our modules in a way that makes the most sense for us as we're building the app.
In production, we want to use the exact same source code structure to generate a single minified, uniquely named file for the JS and the CSS so we can tell the browser to cache those permanently. If we do our job correctly when serving those files, our main application code will be downloaded once per revision of the app.
Moonboots aims to solve this problem. You structure your entire application in the same way that you would in node with Common JS modules requiring each other. It uses browserify under the hood to do all that magic. But then you just configure your client application like so:
Moonboots and Express.js
The full documentation for moonboots is available in the Moonboots repo.
For a preview, here's how we'd configure a clientside moonboots app to be served by Express:
var express = require('express'),
Moonboots = require('moonboots'),
app = express();
// configure our app
var clientApp = new Moonboots({
main: __dirname + '/sample/app/app.js',
developmentMode: false,
libraries: [
__dirname + '/sample/libraries/jquery.js'
],
stylesheets: [
__dirname + '/styles.css'
],
server: app
});
// We also just need to specify the routes at which we want to serve this clientside app.
// This is important for supporting "deep linking" into a single page app. The server
// has to know what urls to let the browser app handle.
app.get('*', clientApp.html());
// start listening for http requests
app.listen(3000);
Moonboots and Hapi
If you're using hapi, there's a version of Moonboots that is structured as a Hapi plugin.
Finding modules
There's a site containing a curated list of npm-installable modules with a quick filter box: humanjs-resources that should help you find things you may need.
Also, there are lots of modules you can find via browserify's module search.
Examples
And Bang
And Bang helps same-page-ify your team with shared tasks and chat.
Many of the tools and approaches of human javascript were extracted from And Bang.
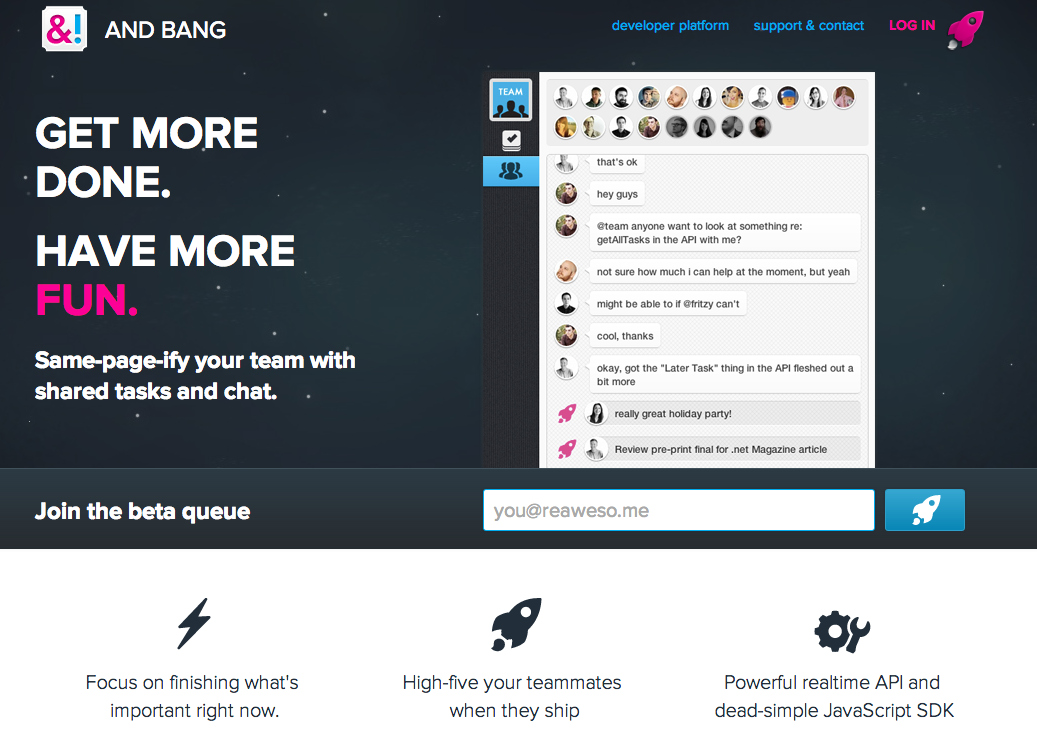
Talky
Talky is truly simple video chat and screen sharing app for groups.
Powered by WebRTC and built on Human Javascript.
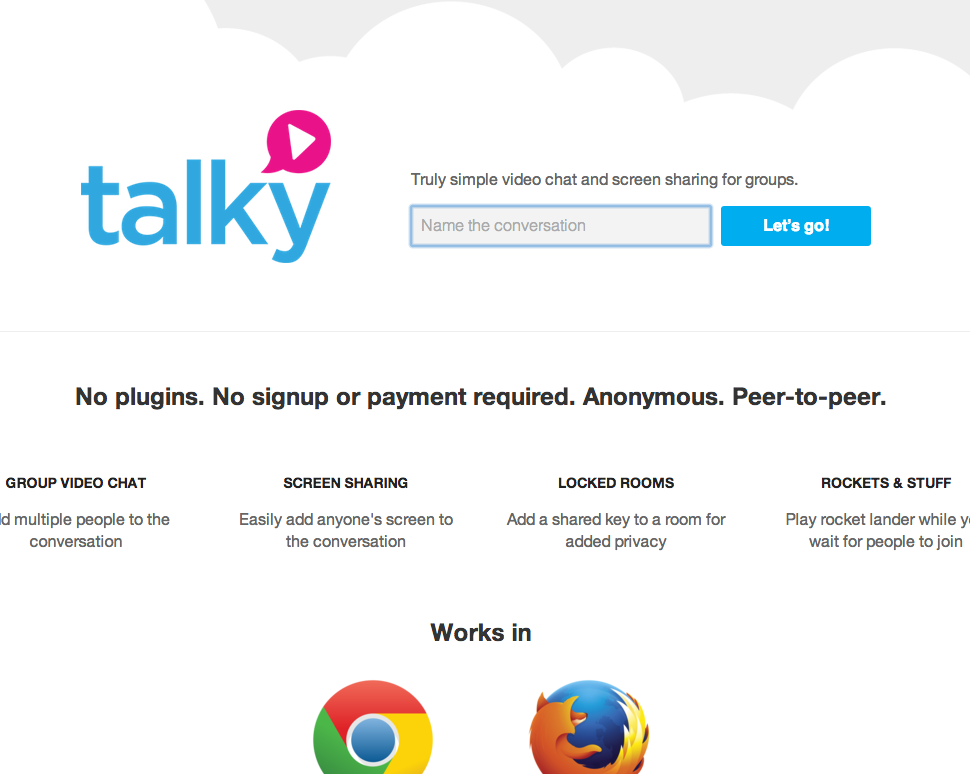
The Resources App
Quick module search of curated modules. The source for this app is available on Github.
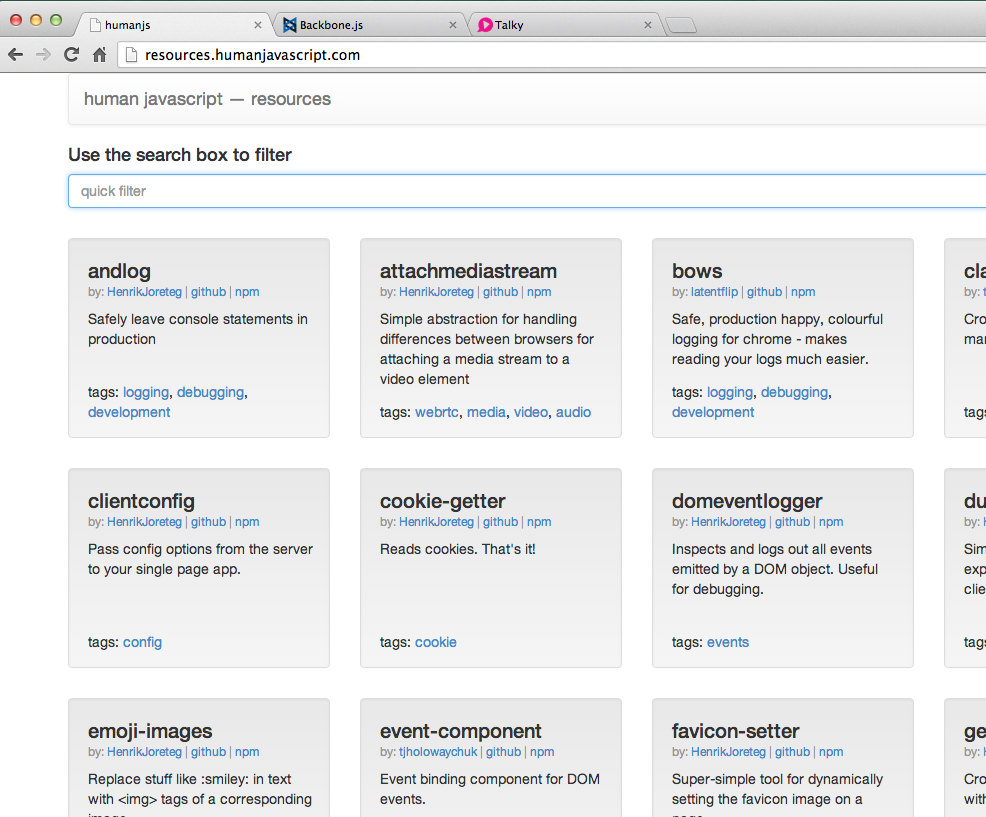
Otalk
Otalk is an open source chat client powered XMPP, stanza.io, and WebRTC. You can think of it as an open source alternative to Skype.
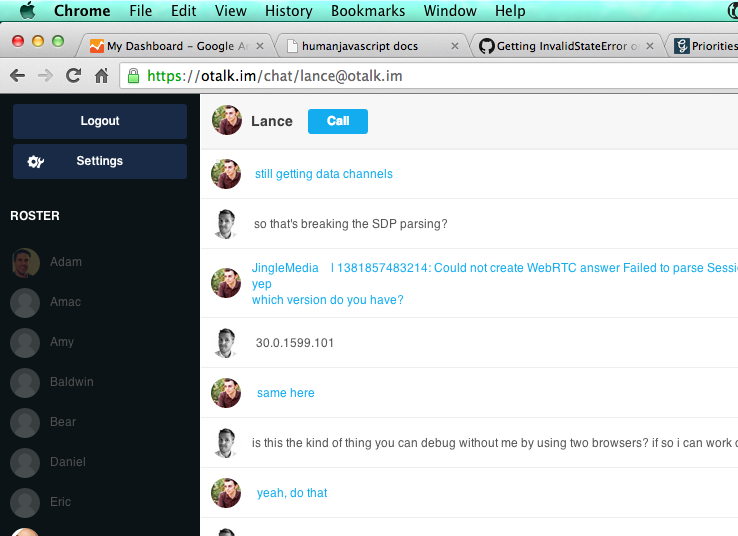
Contributors
Human JavaScript is the combined efforts of lots of people and since all these projects are open source there are simply too many contributors to list.
The project is sponsored by &yet.
The core contributor team consists of:
If you have benefitted from these tools, please consider buying the book or hiring &yet to help with your next development project.